
Enki is a small custom package that gives plugin developers quick and easy access to the Player instance whenever the player starts or stops touching water.
As of the current version, the following can be found within the package:
- enki.constants
- enki.listeners
- enki.players.entity
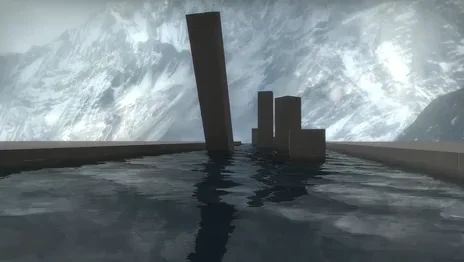
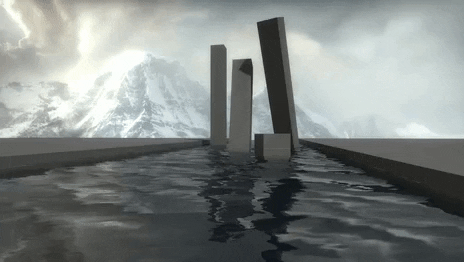
If, for example, you wanted to create a big water splash effect whenever the player initially touches the water (see the first gif):
Syntax: Select all
# ../water_splash/water_splash.py
# Source.Python
from entities.entity import Entity
from stringtables import string_tables
# Enki
from enki.listeners import OnPlayerEnterWater
@OnPlayerEnterWater
def on_player_enter_water(player):
"""Called when a player starts touching water."""
# Did the player enter the water at a reasonable velocity?
if player.velocity.length > 300:
# Let's make a big water splash!
particle = Entity.create('info_particle_system')
particle.origin = player.origin
# NOTE: This particle effect only exists in CS:GO, there are similar
# effects in other games, e.g. 'water_splash_01' in CS:S.
particle.effect_name = 'explosion_basic_water'
particle.effect_index = string_tables.ParticleEffectNames.add_string(
'explosion_basic_water'
)
particle.start()
particle.delay(1, particle.remove)
Syntax: Select all
# ../water_walk/water_walk.py
# Source.Python
from commands import CommandReturn
from commands.client import ClientCommand
from messages import HintText
# Enki
from enki.players.entity import EnkiPlayer
@ClientCommand('+lookatweapon')
def inspect_weapon_pressed(command, index):
"""Called when a player presses their weapon inspect key."""
EnkiPlayer(index).enable_water_walking()
# Tell the player that they can walk on water.
HintText('You can now walk on water.').send(index)
# Block the inspect animation from playing.
return CommandReturn.BLOCK
@ClientCommand('-lookatweapon')
def inspect_weapon_released(command, index):
"""Called when a player releases their weapon inspect key."""
EnkiPlayer(index).disable_water_walking()
HintText('You can no longer walk on water!').send(index)
return CommandReturn.BLOCK
You can find more examples in the repository.

Latest release